Cheat sheet
Just a bunch of short expressions in Swift.
let
- readonly var
println(“ \() “)
?
- Optional (can be not set)
enum Optional<T> { case None case Some(T) }
!
- unwrap an optional (will crash if nil)
all properties have to be initialized and optionals are nil by default
getters and setters
var name:type { get { } set { newValue } }
function signature shortcuts
{ (op1: Double, op2: Double) -> Double in return op1 * op2 } { (op1, op2) in op1 * op2 } { $0 * $1 }
array and map definition shortcuts
Array<Double> - [Double] Dictionary<String, Double> - [String:Double]
Only classes are passed by reference (not structs)
Printable protocol:
var description: String { get { } }
final
prevents subclasses from using override
observe changes to a property willSet
and didSet
{newValue oldValue}
a property can be lazy
required & convenience init
init?(…)
is a failable init
casting as or as? (will not crash)
if let buttonItem = item as? UIButton { … }
preferredFontForTextStyle
set contentMode
to properly redraw on view bounds change
add @IBDesignable
to a view to allow Xcode to draw it and @IBInspectable
to allow to modify properties from Xcode
use class in protocol to allow only classes (and not structs or enums) to implement it
??
if left side is nil use right side
fallthrough
in switch means execute the next case
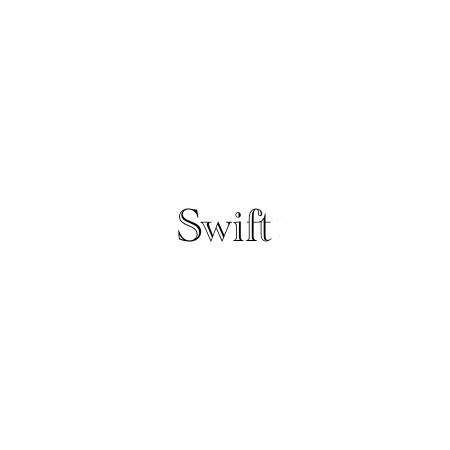
Subscribe to The infinite monkey theorem
Get the latest posts delivered right to your inbox