Catching an exception in Swift using functions!
Recently we had a need in a general way to catch calls that can throw an exception.
Recently we had a need in a general way to catch calls that can throw an exception.
Like with any functional or semi-functional language it is fairly easy to create weird looking constructs with Swift.
Let’s create one but first a function that simply throws an error:
func throwAnError() throws -> Void {
throw DivisionError.ByZero
}
If we call throwAnError()
it must be surrounded by a do...catch
block.
do {
try throwAnError()
} catch let error as NSError {
print("Unable to divide by zero:", error)
} catch {
print("Failed miserably and have no idea what happened.")
}
And it is all great until we have to call throwAnError()
many many times in many different places…
For that we can create a function that will do that for us!
func printError(completion: () throws -> Void)
{
do {
try completion()
} catch let error as NSError {
print("Unable to divide by zero:", error)
} catch {
print("Failed miserably and have no idea what happened.")
}
}
And now we can simply run it as a block:
printError({
try throwAnError()
})
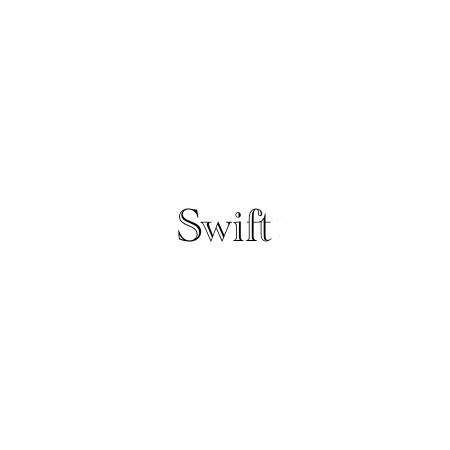
Subscribe to The infinite monkey theorem
Get the latest posts delivered right to your inbox