UIScrollView with AutoLayout, programmatically
It is fairly easy to use UIScrollView with the AutoLayout on iOS 12+ but only if one follows the following steps ...
It is fairly easy to use UIScrollView with the AutoLayout on iOS 12+ but only if one follows the following steps:
- Add UIScrollView and pin it 0,0,0,0 to superview (or your desired size)
NSLayoutConstraint.activate([
scrollView.topAnchor.constraint(equalTo: view.safeAreaLayoutGuide.topAnchor),
scrollView.bottomAnchor.constraint(equalTo: view.safeAreaLayoutGuide.bottomAnchor),
scrollView.leadingAnchor.constraint(equalTo: view.safeAreaLayoutGuide.leadingAnchor),
scrollView.trailingAnchor.constraint(equalTo: view.safeAreaLayoutGuide.trailingAnchor)
])
- Add UIView in ScrollView, pin it 0,0,0,0 to all 4 sides.
NSLayoutConstraint.activate([
contentView.topAnchor.constraint(equalTo: scrollView.contentLayoutGuide.topAnchor),
contentView.bottomAnchor.constraint(equalTo: scrollView.contentLayoutGuide.bottomAnchor),
contentView.leadingAnchor.constraint(equalTo: scrollView.contentLayoutGuide.leadingAnchor),
contentView.trailingAnchor.constraint(equalTo: scrollView.contentLayoutGuide.trailingAnchor)
])
- Center contentView X and Y and set Y’s priority to low. Set contentView height to greaterThanOrEqualTo it’s parent height.
let contentViewCenterY = contentView.centerYAnchor.constraint(equalTo: scrollView.centerYAnchor)
contentViewCenterY.priority = .defaultLow
let contentViewHeight = contentView.heightAnchor.constraint(greaterThanOrEqualTo: view.heightAnchor)
contentViewHeight.priority = .defaultLow
NSLayoutConstraint.activate([
contentView.centerXAnchor.constraint(equalTo: scrollView.centerXAnchor),
contentViewCenterY,
contentViewHeight
])
- Add all views that you need into this view. Don’t forget to set the bottom constraint on the lowest view.
The source code can be found here: seifeet/SwiftFormScrolling
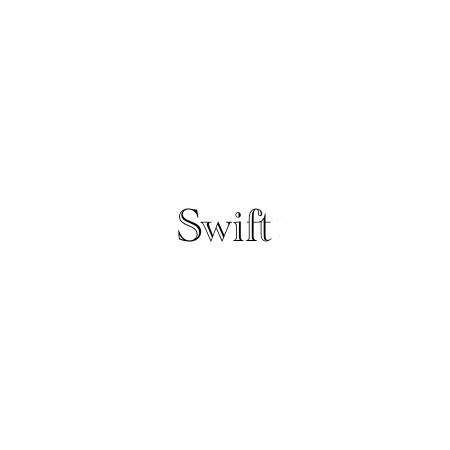
Subscribe to The infinite monkey theorem
Get the latest posts delivered right to your inbox